The Delphi Icon serves as an important element of a Delphi application. This tutorial aims to provide an in-depth understanding of how to create and manage a system tray icon through a Delphi application. Known as the notification area on Windows 2000 upward, this is a space beside the clock where these icons appear. This feature allows for greater user interactivity and application accessibility.
Target Audience for This Tutorial
This tutorial is designed for individuals with a foundational understanding of Delphi. It presupposes that you are familiar with the following:
- Creating procedures, including the necessity of declaring them in both the interface and implementation sections;
- Basic comprehension of form events, such as FormCreate and FormDestroy, and how to utilize them;
- Ability to incorporate controls onto a form using the Delphi IDE.
Step-by-step Tutorial
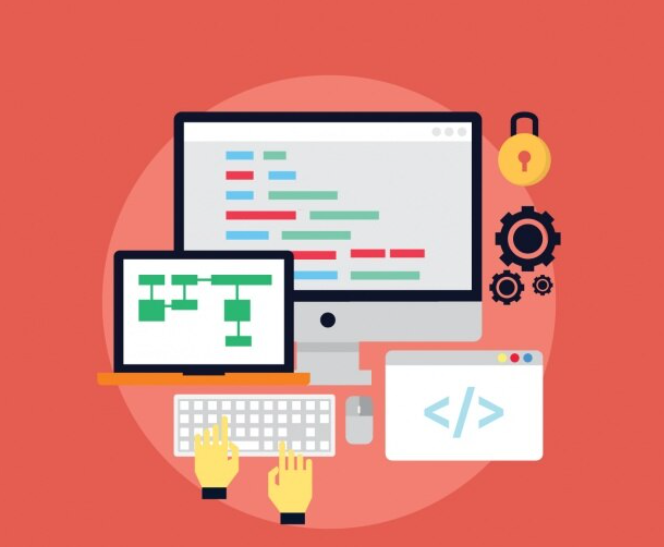
Throughout this guide, the term “System Tray” is used to denote the area beside the clock on Windows 2000 and later versions, which houses icons. It’s worth noting that this area may also be referred to as the Notification Area.
STEP 1
We kick off with a clean slate. Initiate a new application via File -> New -> Application
STEP 2
Proceed to insert the following two variables into the private section of TForm1:
{ Private declarations }
FIconDisplayed: Boolean;
FSystemTrayIconData: TNotifyIconData;
- FIconDisplayed will be set to true following the display of the icon. This variable helps us avoid redundant displays of the icon;
- FSystemTrayIconData encompasses the icon itself. As the TNotifyIconData type is defined within the ShellApi unit, make sure to include this unit in your uses clause.
STEP 3
This step involves the creation and display of the system tray icon, marking it as one of the more complex steps. Pay attention closely as a new procedure named DisplayTrayIcon is introduced, responsible for placing an icon in the system tray.
procedure TForm1.DisplayTrayIcon;
begin
if not FIconDisplayed then
begin
with FSystemTrayIconData do
begin
uID := 1;
Wnd := Handle;
cbSize := SizeOf(FSystemTrayIconData);
hIcon := Application.Icon.Handle;
uCallbackMessage := WM_ICONREPLY;
uFlags := NIF_TIP + NIF_MESSAGE + NIF_ICON;
StrPCopy(szTip, 'Your system tray icon is ready and at your service!');
end;
Shell_NotifyIcon(NIM_ADD, @FSystemTrayIconData);
FIconDisplayed := True;
end;
end;
The TNotifyIconData variable, FSystemTrayIconData, requires the setup of several intricate properties, which we’ll explore here. Though, if you find them overwhelming, feel free to skim through:
- hIcon – represents the icon’s handle;
- uCallbackMessage – this message is sent to the form when there’s interaction with the icon (e.g., a click);
- szTip – the tooltip displayed when hovering over the icon.
To display the icon, this procedure executes Shell_NotifyIcon with the NIM_ADD flag, alongside the address of our FSystemTrayIconData variable.
Next, we need to declare WM_ICONREPLY. Insert this constant at the beginning of your unit, following the first uses clause but before the type declaration. This custom Windows message is generated upon interacting with the tray icon.
const
WM_ICONREPLY = WM_USER + 1;
Alt: A modern browser window and coding brackets on a pink gradient.
STEP 4
Crafting a procedure to conceal the icon is our next step. Fortunately, this is significantly simpler than the icon creation code. Here it is:
procedure TForm1.ConcealTrayIcon;
begin
if FIconDisplayed then
begin
Shell_NotifyIcon(NIM_DELETE, @FSystemTrayIconData);
FIconDisplayed := False;
end;
end;
This procedure verifies if the icon is displayed and, if so, removes it using Shell_NotifyIcon with the NIM_DELETE flag.
STEP 5
Utilize the Delphi IDE to add a button to the form, and bestow it with a creative caption, going beyond the mundane.
This button, when clicked, will toggle the display of the icon in the system tray – showing it upon the first click and removing it upon the second.
STEP 6
Access the OnClick event by double-clicking the button. This event should invoke the DisplayTrayIcon procedure if the icon is not already shown, and ConcealTrayIcon if it is. Here’s the simple code for it:
procedure TForm1.Button1Click(Sender: TObject);
begin
if FIconDisplayed then
ConcealTrayIcon
else
DisplayTrayIcon;
end;
This logic straightforwardly determines whether to display or hide the tray icon based on its current visibility.
STEP 7
To ensure the application compiles successfully, add the following empty procedure to your project:
procedure IconResponse(var Msg: TMessage); message WM_ICONRESPONSE;
procedure TForm1.IconResponse(var Msg: TMessage);
begin
// This procedure is intentionally left empty for now
end;
This empty procedure is a placeholder that defines the response when the tray icon is interacted with. We will implement the functionality in the subsequent part of this tutorial.
STEP 8
To address a minor issue where the tray icon persists after the application is closed, implement the following:
procedure TForm1.FormDestroy(Sender: TObject);
begin
HideTrayIcon;
end;
Incorporate this code by creating a new OnDestroy event for Form1. This ensures the tray icon is removed when the application is closed, contingent on the icon’s presence in the tray, as explained in Step 4.
Upon completing the tutorial, run the application to see the main form with a “Click Me” button. Clicking this button will toggle the appearance of your icon in the system tray. Hovering over the tray icon will display the tooltip set earlier in the tutorial.
Conclusion
Understanding the Delphi Icon and manipulating system tray icons can significantly enhance user interaction and application accessibility. This guide aims to provide a comprehensive understanding of the Delphi Icon, covering creation, handling, and troubleshooting. Incorporating these practices can transform your application, providing a more intuitive and user-centric experience. Knowledge of these processes is a valuable skill for both beginners and seasoned developers, refining their application development capabilities.